This is a Basic Tutorial on understanding scenes. While the majority of the explanations here still apply, most of this tutorial was meant for Cortex Command circa Build 22 (and other parts are much older, dating back to Build 13).
One can obviously use the map editor included in the game for creation,
but this is for those who want more customizability/understanding, or those who haven't purchased the
game yet.
This tutorial works by giving you a walkthrough of all the variables and their functions.
Here's how it goes:
1. Start Out by making the base code .ini
For this example we are just going to copy
a scene ini from the base and put it in
a folder called *yourname*.rte
2. Once you have all the code you need to
get started... get started.
This defines what objects are going into your
scene (not where they are placed)
Code:
////////////////////////////////////////////////
//Begin list of TerrainObjects
AddTerrainObject = TerrainObject
InstanceName = Brain Vault
// Foreground color bitmap
FGColorFile = ContentFile
Path = Base.rte/Scene/Objects/BrainVaultFG.bmp
// Material bitmap
MaterialFile = ContentFile
Path = Base.rte/Scene/Objects/BrainVaultMat.bmp
// Background color bitmap
BGColorFile = ContentFile
Path = Base.rte/Scene/Objects/BrainVaultBG.bmp
In here it shows the code for a brain vault.
Code:
AddTerrainObject = TerrainObject
InstanceName = Brain Vault
This defines the name of your object.
Code:
FGColorFile = ContentFile
Path = Base.rte/Scene/Objects/BrainVaultFG.bmp
This picture path defines what your foreground will look like.
Code:
MaterialFile = ContentFile
Path = Base.rte/Scene/Objects/BrainVaultMat.bmp
This defines what your object is made of. Look toward the bottom to see the basics of material file editing.
Code:
BGColorFile = ContentFile
Path = Base.rte/Scene/Objects/BrainVaultBG.bmp
This defines the background.
So far, so simple.
Next bit of code defines the debris in your ground (gold and rocks)
Code:
AddTerrainDebris = TerrainDebris
InstanceName = Boulders
DebrisFile = ContentFile
// When loading, 000-001-002 etc is added automatically before .bmp
Path = Base.rte/Scenes/Objects/Boulders/Boulder.bmp
DebrisPieceCount = 103
DebrisMaterial = Material
CopyOf = Stone
TargetMaterial = Material
CopyOf = Earth
OnlyOnSurface = 0
MinDepth = 0
MaxDepth = 300
DensityPerMeter = 1.5
The name is boulders there are 103 in map.
There are 1.5 per 20 pixels.
can only go to a depth of 300 pixels.
Finally you get to name your scene!
Code:
AddScene = Scene
InstanceName = Grasslands
LocationOnPlanet = Vector
X = 0
Y = 0
// Gravity acceleration, m/s^2
GlobalAcceleration = Vector
X = 0
Y = 19.82
The y number is the gravity.
Grasslands is the name. Don't wear it out.
Global Acceleration is the Gravity for the Scene (It's usually frowned upon if you change it).
LocationOnPlanet is where your little dot on the Planet is (Only If this is a Lua Campaign Mission).
Code:
// Terrain SceneLayer
Terrain = SLTerrain
InstanceName = Grasslands Terrain
BitmapFile = ContentFile
Path = Base.rte/Scenes/Grasslands.bmp
WrapX = 1
WrapY = 0
ScrollRatio = Vector
X = 1.0
Y = -1.0
BackgroundTexture = ContentFile
Path = Base.rte/Scenes/Textures/DirtDark.bmp
This is where you need to define a material file for the map.
I'm sure that it uses the same colors as MATs.
Background texture file is the background of the terrain once you knock it loose.
Code:
WrapX = 1
WrapY = 0
ScrollRatio = Vector
X = 1.0
Y = -1.0
This defines where the map wraps (loops).
But you must change the scroll ratios, if you change
the wrap variables to get a map in the 2d dimension.
Code:
/////////////////////////////////////////
// Terrain Debris which is scattered
AddTerrainDebris = TerrainDebris
CopyOf = Gold
AddTerrainDebris = TerrainDebris
CopyOf = Plants
AddTerrainDebris = TerrainDebris
CopyOf = Boulders
This must be here to make your gold and stuff appear in the dirt.
This also uses the boulders we defined earlier.
Code:
/////////////////////////////////////////
// Material frostings
AddTerrainFrosting = TerrainFrosting
TargetMaterial = Material
CopyOf = Topsoil
FrostingMaterial = Material
CopyOf = Grass
MinThickness = 5
MaxThickness = 7
InAirOnly = 1
This is the new way to make grass on dirt.
Make the grass target any material, and then
you can make the grass anything you want.
Min and max thickness is the density (prollabably per
meter (20 pixels)) of the foliage or grass.
InAirOnly is the variable which makes it appear only
in the presence of air (Pink) and the target material.
Code:
/////////////////////////////////////////
// List of TerrainObjects to apply
PlaceTerrainObject = TerrainObject
CopyOf = Rocket Silo
Location = Vector
X = 1357
Y = 693
This defines where your earlier terrain objects are placed.
The y is pixels from the top to the bottom. 0 is top of map.
The x is pixels from the loop to the right. 0 is inside loop.
Code:
PlaceMovableObject = ACRocket
CopyOf = Rocket MK1
Position = Vector
X = 1605
Y = 855
Team = 0
This defines where and what actors are placed.
Setting "Team =" to 1 would make it the enemy Rocket.
Same with the brain. As a matter of fact:
Lord Tim wrote:
You can skip the base placement phase by putting in brains for both players in the scene.ini.
Code:
AddBackgroundLayer = SceneLayer
InstanceName = Middle Layer
DrawTransparent = 1
WrapX = 1
WrapY = 0
ScrollRatio = Vector
X = 0.25
Y = 1200
BitmapFile = ContentFile
Path = Base.rte/Scenes/Backdrops/Middle.bmp
AddBackgroundLayer = SceneLayer
InstanceName = Clouds Layer
DrawTransparent = 1
WrapX = 1
WrapY = 0
ScrollRatio = Vector
X = 0.02
Y = 2400
BitmapFile = ContentFile
Path = Base.rte/Scenes/Backdrops/Clouds.bmp
AddBackgroundLayer = SceneLayer
InstanceName = Sky Layer
DrawTransparent = 0
WrapX = 0
WrapY = 0
ScrollRatio = Vector
X = 640
Y = 1200
BitmapFile = ContentFile
Path = Base.rte/Scenes/Backdrops/Back.bmp
This defines the Sky of your Scene
Default is Rocks from the Horiz-Land scene.
3. Now that the Explaining is over we can start
making the stuff in the map.
MATS:
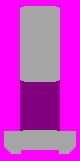
This is an example of a MAT (Material file) that you might find.
Grey = Concrete
Purple = empty space that dirt doesn't go into (Air) or empty space. Also prevents debris when used in terrain.
Pink = is completely empty space. or Air.
These are all of the things needed to make bases so this should be sufficient.
See bonus for custom MATS.
Contours/Map MATsCopy a map that has already been made like Grass Lands or Horizland.
And make the open air all that hot pink color.
And draw your own map. You can use custom materials by using the method below.
And make sure to use RLE compression otherwise errors will befall you.
But if you use paint and copy someone else's map for colors and stuff,
use that image and redraw terrain for your own uses. That way it will maintain
RLE compression. Or you don't need it; all you really need is the palette.
Blah:
http://www.google.com/search?q=RLE+CompressionTip: Make it look natural not just a map made of topsoil.
4. LUA! I'm sure this is the part you have all been waiting for.
Here's how to incorporate a written Lua script into your scene (not how to write lua, silly!)
Add your .lua file into your scene folder, and at the end of your *Scene Here*.ini add:
Code:
AddActivity = GAScripted
PresetName = *Your Scene*
SceneName = *Your Scene Again*
ScriptFile = *Your Mod*.rte/*Your Lua*.lua
InCampaignStage = 1 // <-- Critical That you have this part. It needs to be set to one.
LuaClassName = *Whatever LuaClassName you used in the .lua file*
TeamCount = 2 // <-- Probably always 2
PlayerCount = 1 //Change to How many players you intend to have use this map.
TeamOfPlayer1 = 0 // Player 1's Team (Red)
TeamOfPlayer2 = 1 // Player 2's Team (Green)
You also need to change the "Location on Planet Vector" at the beggining of your scene to where
you want the little dot on the planet to be located.
Now you can mess with your Lua file, which is a whole 'nother thing.
BONUS (Not Sure if this works anymore):
To make custom MATS you can make a MOSRotating
that is made out of custom material the let loose on a map.
Wait till' it becomes static/terrain and then press "Ctrl+m" twice.
Then look at the color of your object. Then press Print Screen.
Go into paint and take the color of the object and paste it into
your MAT. Now you can make fully custom terrain objects.
Happy map making!